Quantum computing is a rapidly evolving field that promises to revolutionize the way we solve complex problems. One of the most powerful tools available for quantum computing enthusiasts and professionals is Qiskit, an open-source quantum computing framework developed by IBM. In this post, we’ll explore what Qiskit is, how to use it to create quantum circuits, and provide a detailed example of creating Bell states, which are fundamental for quantum teleportation.
What is Qiskit?
Qiskit is an open-source software development kit (SDK) for working with quantum computers. It provides tools to create and manipulate quantum programs and run them on prototype quantum devices. Qiskit aims to make quantum computing accessible to a broad audience, from researchers and developers to students and educators.
Key Components of Qiskit
Qiskit consists of several key components:
- Qiskit Terra: The foundation for composing circuit and pulse-level quantum programs.
- Qiskit Aer: High-performance simulators for quantum circuits.
- Qiskit Ignis: Tools for quantum hardware verification, noise characterization, and error correction.
- Qiskit Aqua: Algorithms for quantum computing applications in fields like chemistry, AI, optimization, and finance.
Getting Started with Qiskit
To start using Qiskit, you need to install it. If you haven’t already, you can install Qiskit using pip:
pip install qiskit
Creating Quantum Circuits with Qiskit
A quantum circuit is a sequence of quantum gates, measurements, and possibly resets, which operate on a quantum register (a set of qubits).
Here, we’ll provide a detailed example of how to create Bell states using Qiskit. Bell states are specific quantum states of two qubits representing the simplest and most powerful forms of entanglement, making them essential for quantum teleportation.
Example: Creating Bell States
Below is the Python code to create and visualize the four Bell states using Qiskit.
from qiskit import QuantumCircuit, Aer, execute
from qiskit.visualization import plot_state_city, plot_bloch_multivector
import matplotlib.pyplot as plt
def create_bell_state(state):
qc = QuantumCircuit(2)
# Create the Bell state |Φ+⟩ = (|00⟩ + |11⟩) / sqrt(2)
qc.h(0) # Apply Hadamard gate to the first qubit
qc.cx(0, 1) # Apply CNOT gate with control qubit 0 and target qubit 1
if state == 'phi_minus':
qc.z(0) # Apply Z gate to the first qubit to create |Φ-⟩
elif state == 'psi_plus':
qc.x(0) # Apply X gate to the first qubit to create |Ψ+⟩
elif state == 'psi_minus':
qc.x(0) # Apply X gate to the first qubit
qc.z(0) # Apply Z gate to the first qubit to create |Ψ-⟩
return qc
# List of Bell states
bell_states = ['phi_plus', 'phi_minus', 'psi_plus', 'psi_minus']
# Backend to simulate the quantum circuit
backend = Aer.get_backend('statevector_simulator')
# Create and plot each Bell state
for state in bell_states:
qc = create_bell_state(state)
result = execute(qc, backend).result()
statevector = result.get_statevector()
print(f"\nBell State |{state}⟩")
print(statevector)
plot_state_city(statevector)
plt.title(f"Statevector of Bell State |{state}⟩")
plt.show()
plot_bloch_multivector(statevector)
plt.title(f"Bloch Sphere of Bell State |{state}⟩")
plt.show()
Explanation of the Code
- Imports:
QuantumCircuit
is used to create quantum circuits.Aer
andexecute
are used to run the quantum circuits on a simulator backend.plot_state_city
andplot_bloch_multivector
are used for visualizing the state vector and Bloch sphere representation of the qubits.
- Creating Bell States:
- The function
create_bell_state
takes a state name as input and creates the corresponding Bell state. - For ∣Φ+⟩∣Φ+⟩, we apply a Hadamard gate (H) to the first qubit followed by a controlled-NOT gate (CX) with the first qubit as the control and the second qubit as the target.
- For the other Bell states, additional gates (X and/or Z) are applied to modify ∣Φ+⟩∣Φ+⟩ to the desired Bell state.
- The function
- Simulating and Visualizing:
- We loop through the list of Bell states, create each state using the
create_bell_state
function, and simulate it using the statevector simulator backend. - The resulting statevector is printed and visualized using
plot_state_city
andplot_bloch_multivector
for better understanding.
- We loop through the list of Bell states, create each state using the
Conclusion
Qiskit is a powerful tool for exploring quantum computing. By using Qiskit, you can create and manipulate quantum circuits, simulate their behavior, and visualize quantum states. The example demonstrates how to create and visualize Bell states, which are fundamental in many quantum information processes, including quantum teleportation.
Stay connected with MilovanInovation for more insights and tutorials on quantum computing and other cutting-edge technologies!
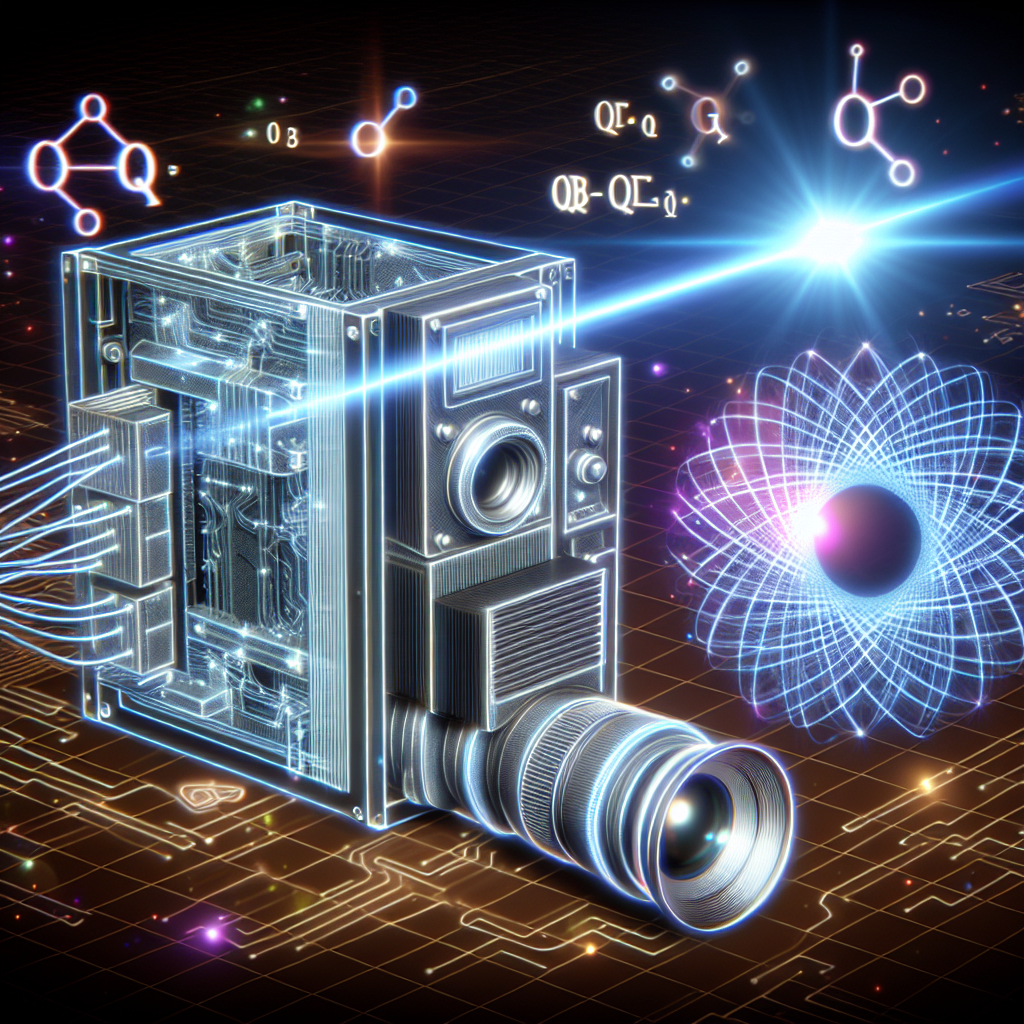
Leave a Reply